Receipts
DATA = JSON.parse('{
"receipt_id":"meow0101021",
"items":[
{
"id":"100",
"name":"Classic t-shirt wit Kitten",
"currency":"USD",
"price":"15.00",
"thumb_url":"http://www.comedyvsnerds.com/assets/cvn_transparent-022819895eb48d3a76ee6627b1a6cda2.png",
"item_url":"http://www.comedyvsnerds.com/?item_id=100",
"quantity":"2",
"metadata":{
"brand":"Awesome Comedy Show",
"size":"L",
"color":"White",
"fun": "A lot!"
}
}
],
"timestamp":"1423076008",
"account_holder_name":"Matt Berman",
"recipient_name":"Matt Berman",
"structured_address":{
"street_1":"25 Taylor str",
"city":"San Francisco",
"state":"CA",
"postal_code":"94133",
"country":"US"
},
"shipping_method":"USPS",
"payment_method":"Visa 1234",
"subtotal":"30.00",
"shipping_cost":"5.00",
"total_tax":"3.00",
"total_cost":"38.00",
"currency":"USD",
"order_url":"http://www.comedyvsnerds.com?orderID=order123456789",
"cancellation_url":"http://www.comedyvsnerds.com?cancelID=order123456789"
}')
TYPE = 'retail_receipts'
Name | Description | Type | Required |
---|---|---|---|
receipt_id | unique order number | string | Y |
items | array of items being ordered | array | Y |
timestamp | order placed time | timestamp | Y |
account_holder_name | name of the person who placed the order | string | Y |
receipt_name | name of the person receiving the shipment item | string | Y |
structured_address | structured shipping address | shipping | Y |
shipping_method | shipping carrier (UPS/Fedex etc) | string | N |
payment_method | should be card name and last 4 digits (Visa & 1234) | string | Y |
subtotal | order subtotal amount | float | Y |
shipping_cost | total shipping amount | float | Y |
total_tax | tax amount | float | Y |
total_cost | total order amount | float | Y |
currency | pricing currency (ex: USD) | enum | Y |
order_url | URL of order details page | URL | N |
status values: PREPARED_SHIP, PARTIALLY_SHIPPED, SHIPPED, DELIVERED, EMPTY_STRING, CANCELED
items Object:
Name | Description | Type | Required |
---|---|---|---|
id | unique product number | string | Y |
name | item name | string | Y |
currency | item pricing currency e.g. USD | string | Y |
price | item price | string | Y |
thumb_url | item image to be displayed | URL | N |
item_url | URL for item | URL | Y |
quantity | item quantity | string | N |
metadata | item metadata that will display in receipts (see details below) | metadata | N |
metadata Object:
Name | Description | Type | Required |
---|---|---|---|
brand | item brand name | string | N |
size | item size (please add "Size " before numeric sizes, e.g. 4, 2T, etc.) | string | N |
color | item color description | string | N |
gender | gender for item | enum (M/F or "" for gender neutral) | N |
structured_address Object:
Name | Description | Type | Required |
---|---|---|---|
street_1 | street address 1 | string | Y |
street_2 | street address 2 | string | N |
city | city name | string | Y |
state | 2 character state name | state name | Y |
postal_code | 5 digit postal code | string | Y |
country | 2 character country name. ISO3166 alpha-2 | string | Y |
Shipment Notifications
DATA = JSON.parse('{
"receipt_id":"meow0101021",
"carrier":"UPS",
"tracking_number":"1ZW098R40361231305",
"carrier_tracking_url":"http://www.ups.com/WebTracking/processInputRequest?tracknum=1ZW098R40361231305",
"items":[
{
"id":"100",
"quantity":"2"
}
],
"ship_date":"1427824997",
"origin":{
"street_1":"",
"street_2":"",
"city":"Cleveland",
"state":"OH",
"postal_code":"44101",
"country":"US"
},
"destination":{
"street_1":"1 Hacker Way",
"street_2":"",
"city":"Menlo Park",
"state":"CA",
"postal_code":"94025",
"country":"US"
},
"weight":"4.5",
"weight_unit":"LBS"
}')
TYPE = 'retail_shipments'
Name | Description | Type | Required |
---|---|---|---|
receipt_id | unique existing order number | string | Y |
carrier | shipping carrier enumerated values e.g. UPS | enum (see below) | Y |
tracking_number | carrier unique/valid tracking number (required for UPS and USPS) | string | Y |
carrier_tracking_url | URL of shipment tracking site | string | N |
items | array of items being shipped | items[] (see below) | Y |
ship_date | date/time of shipment | timestamp | Y |
origin | structured address of shipment origin | origin (see below) | Y |
destination | structured address of shipment destination | destination (see below) | Y |
weight | shipment numeric weight (default value = 1) | float | N |
weight_unit | shipping weight unit (default value = LBS) | enum (see below) | N |
carrier Values:
UPS
FedEx
USPS
[all other carriers (DHL, OnTrac, etc.) should be returned in a human-readbable form, as this is what will be passed through to the buyer.]
items Object:
Name | Description | Type | Required |
---|---|---|---|
id | unique product number | string | Y |
quantity | item quantity | string | Y |
origin Object:
Name | Description | Type | Required |
---|---|---|---|
street_1 | street address 1 | string | N |
street_2 | street address 2 | string | N |
city | city name | string | Y |
state | 2 character state name | state name | Y |
postal_code | 5 digit postal code | string | Y |
country | 2 character country name. ISO3166 alpha-2 | string | Y |
destination Object
Name | Description | Type | Required |
---|---|---|---|
street_1 | street address 1 | string | Y |
street_2 | street address 2 | string | N |
city | city name | string | Y |
state | 2 character state name | state name | Y |
postal_code | 5 digit postal code | string | Y |
country | 2 character country name. ISO3166 alpha-2 | string | Y |
weight_unit Values (case sensitive):
LBS
KGS
Order Update
DATA = JSON.parse('{
"receipt_id":"meow0101021",
"update_id":"update2",
"update_type":"update_quantity",
"items":[
{
"id":"100",
}
],
"timestamp":"1428444852",
"updated_sub_total":"20.00",
"updated_shipping_cost":"5.00",
"updated_total_tax":"2.00",
"updated_total":"27.00",
"currency":"USD"
}')
TYPE = 'retail_updatereceipts'
If updated_sub_total, updated_shipping_cost, updated_total_tax, or updated_total is omitted, the modified receipt will contain the corresponding value as previously POSTed to retail_receipts and/or /retail_updatereceipts
Fields:
Name | Description | Type | Required |
---|---|---|---|
receipt_id | unique existing order number | string | Y |
update_id | optional unique identifier of this update | string | N |
update_type | cancel_item or update_quantity | enum | Y |
items | array of items being updated | items[] (see below) | Y |
timestamp | order update time | timestamp | Y |
updated_sub_total | updated order subtotal amount | float | N |
updated_shipping_cost | updated total shipping amount | float | N |
updated_total_tax | updated tax amount | float | N |
updated_total | updated total order amount | float | N |
currency | pricing currency e.g. USD | string | N |
update_type values:
cancel_item, update_quantity
items Object:
Name | Description | Type | Required |
---|---|---|---|
id | unique product number | string | Y |
quantity | updated item quantity | string | N |
General Notifications
If you have customers interested in one or more items that are out of stock, they can get notified when an item is available. You can add this as a link in your site labeled Notify Me and make a call the API when an item is in stock.
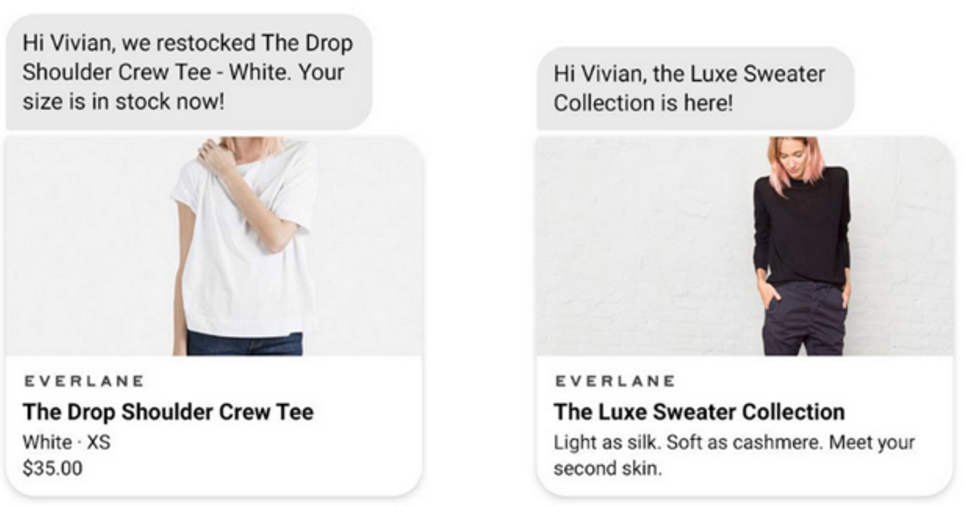
When you add this plugin, you can configure the URL that the customer goes to after they click Notify Me. You can take someone to the page for the item, or a shopping cart with that item already loaded, and so on.
DATA = JSON.parse('{
"name":"New Comedy VS Nerds Show",
"notification_id":"product123456789",
"url":"http://www.google.com",
"thumb_url":"http://www.google.com/share5.png",
"category":"retail_item",
"currency":"USD",
"price":"35.00",
"desc":"Awesome Comedy VS Nerds comedy show, starring Loui CK and Bruce Williams",
"metadata":{
"brand":"ComedyVSNerds",
"kitten":"true",
"funny":"veery"
}}')
TYPE = 'notifyme'
Fields
Name | Description | Type | Req |
---|---|---|---|
notification_id | unique product number | string | Y |
name | name of the product | string | Y |
url | link of the product on your website | URL | Y |
thumb_url | product image to be displayed | URL | Y |
category | Product category | enum | Y |
currency | Pricing currency e.g. USD | enum | N |
price | Price of the product in the above currency | string | N |
desc | Description of the product | string | N |
metadata | Other details about the product | metadata | N |
Metadata Object:
Name | Description | Type | Required |
---|---|---|---|
brand | item brand name | string | N |
size | item size (please add "Size " before numeric sizes, e.g. 4, 2T, etc.) | string | N |
color | item color description | string | N |